PROJECT: MeNUS
This portfolio serves as a summary of my contributions for MeNUS.
Overview
MeNUS is an all-in-one restaurant management desktop application developed to help restaurant owners and managers streamline the process of managing restaurants. It allows the restaurant owners and managers to control their inventory, menu, sales and reservations efficiently all-in-one place. MeNUS is also designed to help restaurants save cost from running expensive and independent systems.
Summary of Contributions
-
Code contributed: RepoSense
-
Major enhancement: Added Menu Management feature
-
What it does: Enables the user to manage the menus with the various menu management commands.
-
Justification: It can be messy and tedious to manage menus manually. This feature helps users to streamline the process of managing menus by enabling them to modify the menus efficiently with just a single command.
-
Highlights: This enhancement integrates with some existing sales and ingredients management commands. It requires an in-depth analysis and understanding of MeNUS architecture to ensure that commands of each management feature is integrated properly together.
-
-
Minor enhancement: Added animation to the GUI (Pull request #214)
-
What it does: Plays the FadeTransition for a short duration when the user enters a command.
-
-
Other contributions:
-
Project management:
-
Maintained issue tracker
-
Managed the integration of the features for MeNUS. Ensured that each feature was properly integrated into MeNUS.
-
-
Enhancements to existing features:
-
Updated the JavaFX Cascading Style Sheets(CSS) theme (Pull request #178)
-
-
Documentation:
-
Added user stories, use cases and instructions for manual testing in the Developer Guide: #37, #114, #142, #282
-
Added menu management features description in User Guide and implementation in Developer Guide
-
-
Community:
-
Contributions to the User Guide
The following sections below showcase extracts of my contributions to the User Guide and my ability to write documentation targeting end-users. |
Menu Management
Adding an item to the menu: add-item
or ai
Adds an item to the menu
Format: add-item n/ITEM_NAME p/ITEM_PRICE [t/TAG]…
Examples:
-
add-item n/Burger p/2
-
ai n/Burger Set p/4.5 t/Set
Editing an item in the menu : edit-item
or ei
Edits an existing item in the menu.
Format: edit-item INDEX [n/ITEM_NAME] [p/ITEM_PRICE] [t/TAG]…
Examples:
-
edit-item 1 n/burger p/3
Edits the name and price of the 1st item to beburger
and3
respectively. -
ei 2 p/4 t/
Edits the price of the 2nd item to be4
and clears all existing tags.
Deleting an item by index: delete-item-index
or dii
Deletes the specified item from the menu.
Format: delete-item-index INDEX [ei/INDEX]
Examples:
-
list-items
delete-item-index 2
Deletes the 2nd item in the menu. -
fi Cheese
dii 1 ei/3
Deletes 1st item, 2nd item and 3rd item in the results of thefind-item
command.
Deleting an item by name: delete-item-name
or din
Deletes the specified item from the menu.
Format: delete-item-name ITEM_NAME
Examples:
-
delete-item-name Apple Juice
Deletes theApple Juice
item from the menu. -
din Cheese Fries
Deletes theCheese Fries
item from the menu.
Filter menu by tag: filter-menu
or fm
Finds items whose tags match any of the given keywords.
Format: filter-menu KEYWORD [MORE_KEYWORDS]…
KEYWORD for filter-menu must be alphanumeric
|
Examples:
-
filter-menu monday
Returns any item that contains tagmonday
-
fm set monday
Returns any item that contains tagset
ormonday
Giving a discount to an item : discount-item
or dci
Gives the item identified by the index number used in the displayed item list a discount.
Format: discount-item INDEX|ALL [ei/INDEX] dp/PERCENTAGE
Examples:
-
list-items
discount-item 2 dp/20
Give the 2nd item in the menu a 20% discount. -
fi Cheese
dci ALL dp/0
Revert all items in the results of thefind-item
command to original price. -
li
dci 1 ei/3 dp/50
Give the 1st item to the 3rd item in the menu a 50% discount.
Contributions to the Developer Guide
The following sections below showcase extracts of my contributions to the Developer Guide. They also showcase my ability to write technical documentation and the technical depth of my contributions to the project. |
Menu management feature
Menu management feature extends MeNUS
with a menu and provides the users with the ability to add items to the
menu, edit items and remove items from the menu.
Current Implementation
The menu is stored internally as items
, which is a UniqueItemList
object that contains a list of Item
objects.
The menu management feature implements the following operations:
-
add-item
command — Adds an item to the menu. The item must not already exist in the menu. -
edit-item
command — Replaces the target item with the editedItem. Target item must be in the menu and editedItem must not be the same as another existing item in the menu. -
delete-item-index
ordelete-item-name
command — Removes the equivalent item(s) from the menu. The item(s) must exist in the menu. -
list-items
command — Lists all the items in the menu. -
clear-menu
command — Removes all items from the menu. -
select-item
command — Selects an item in the menu and loads the page of the selected item. -
sort-menu
— Sorts the menu by name or price. The user must specify the sorting method. -
find-item
command — Finds items whose names contain any of the given keywords. -
filter-menu
command — Finds items whose tags contain any of the given keywords. -
today-special
command — Finds items whose tags contain theDAY_OF_THE_WEEK
. -
discount-item
command — Gives discount to the equivalent item(s) in the menu. The item(s) must exist in the menu. -
recipe-item
command — Adds a recipe to the equivalent item in the menu. The item must exist in the menu. -
add-required-ingredients
command — Adds the required ingredients to the equivalent item in the menu. The item must exist in the menu.
Each Item
object consists of Name
, Price
, Recipe
, Set<Tag>
and Map<IngredientName, Integer>
sort-menu
Command
The sort-menu
command is facilitated by SortMenuCommand
and SortMenuCommandParser
. The command takes in
user input for the sorting method.
Currently only sort by name or price |
The SortMenuCommandParser
will parse the user input and checks if the input is valid. If the input is valid, it
constructs an SortMenuCommand
object.
If the input is not valid, it will throw a ParseException
|
The SortMenuCommand
object will indirectly call the UniqueItemList#sortItemsByName()
or
UniqueItemList#sortItemsByPrice()
and the sorts the menu.
After sorting the menu, it will save the current state for undo/redo.
The following activity diagram summarizes what happens when a user executes sort-menu
command:
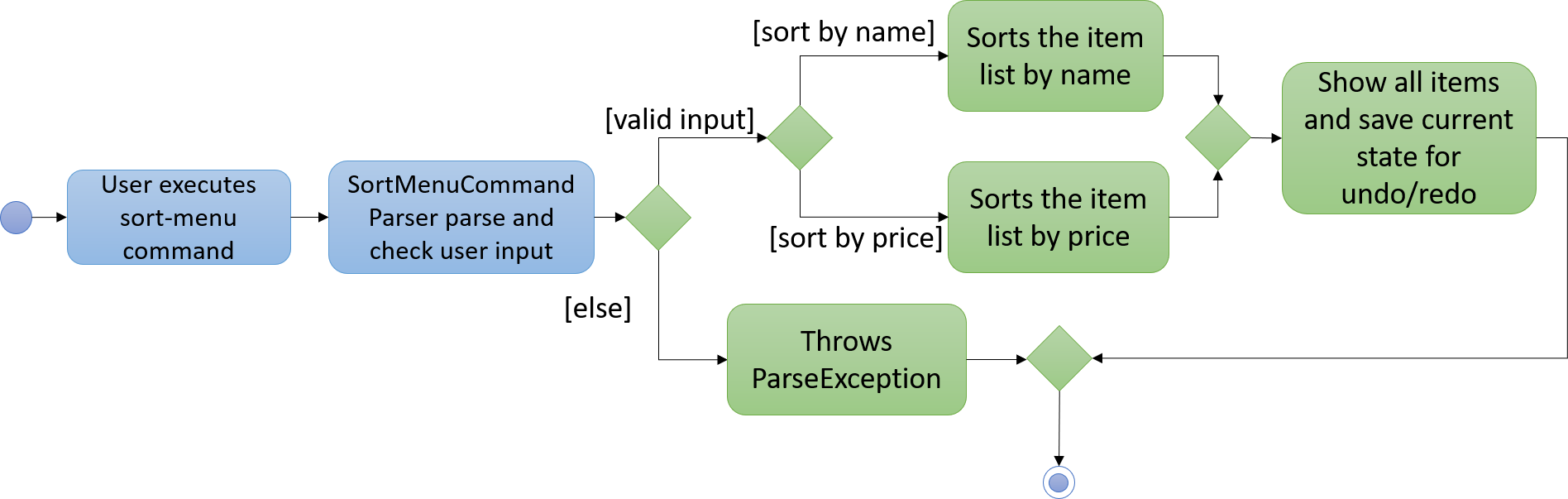
Figure 4.8.2.1: SortMenu Activity diagram
The following sequence diagram shows how the sort-menu
command works:
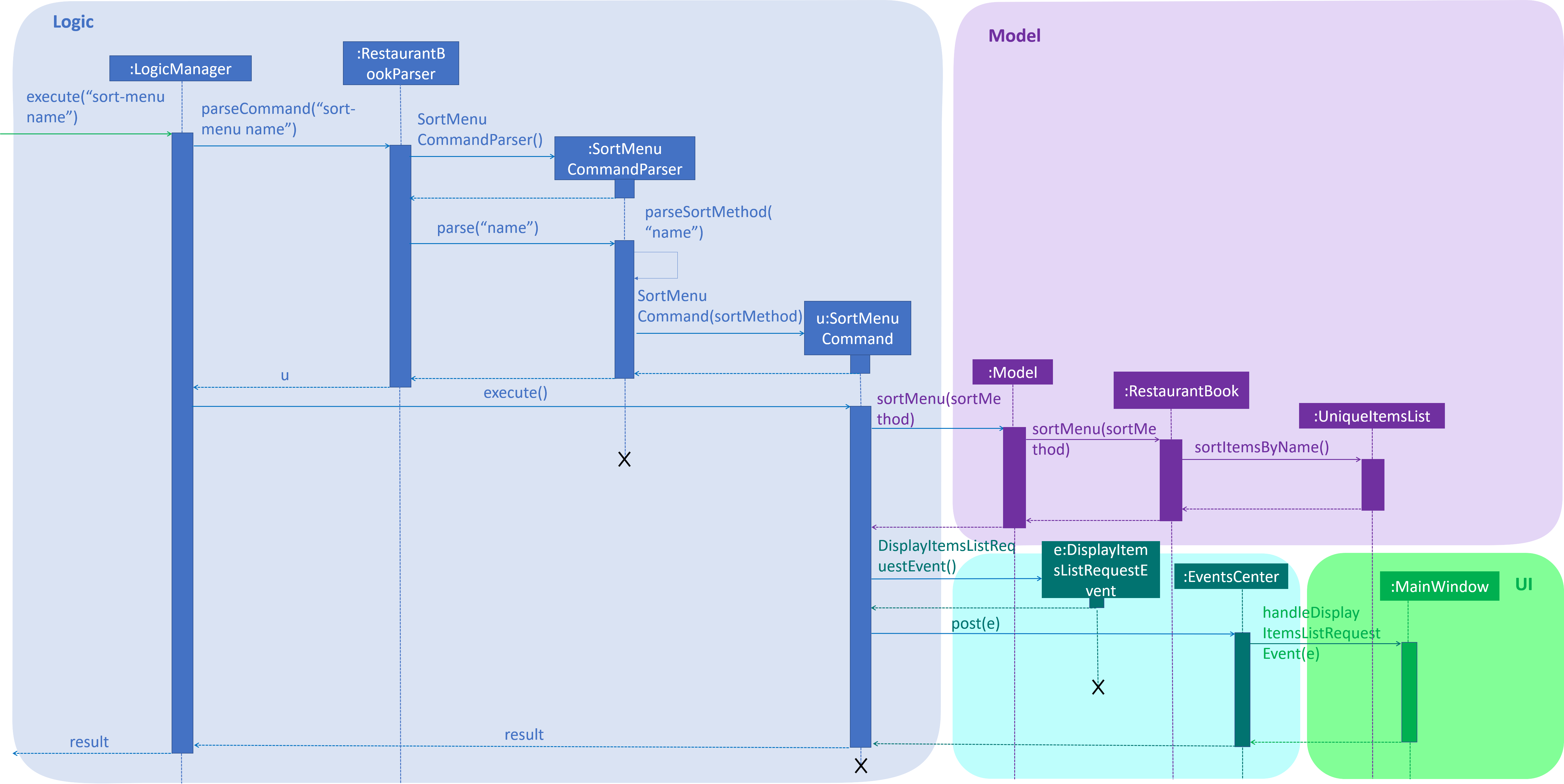
Figure 4.8.2.2: SortMenu Sequence diagram
discount-item
Command
The discount-item
command is facilitated by DiscountItemCommand
and DiscountItemCommandParser
. The command
takes in user input for discount percentage and index or the keyword all
.
The DiscountItemCommandParser
will parse the user input and checks if the input is valid. It will check if the
keyword all
is entered. If keyword all
is entered, DiscountItemCommandParser
will construct an
DiscountItemCommand
object with isAll
set to true. If index or a range of index is entered, it will construct an
DiscountItemCommand
object with isAll
set to false.
If the inputs are not valid, it will throw a ParseException
|
The DiscountItemCommand
object will check if isAll
is true. If isAll
is true, it will give the discount to all
items in the UniqueItemList
. Otherwise, it will give the discount to the item(s) specified by the index or the
range of index. The DiscountItemCommand
object create an Item with discounted price
and indirectly call
UniqueItemList#setItem(Item target, Item editedItem)
to update the Item
with Item with discounted price
. It will
then save the current state for undo/redo.
The following activity diagram summarizes what happens when a user executes discount-item
command:
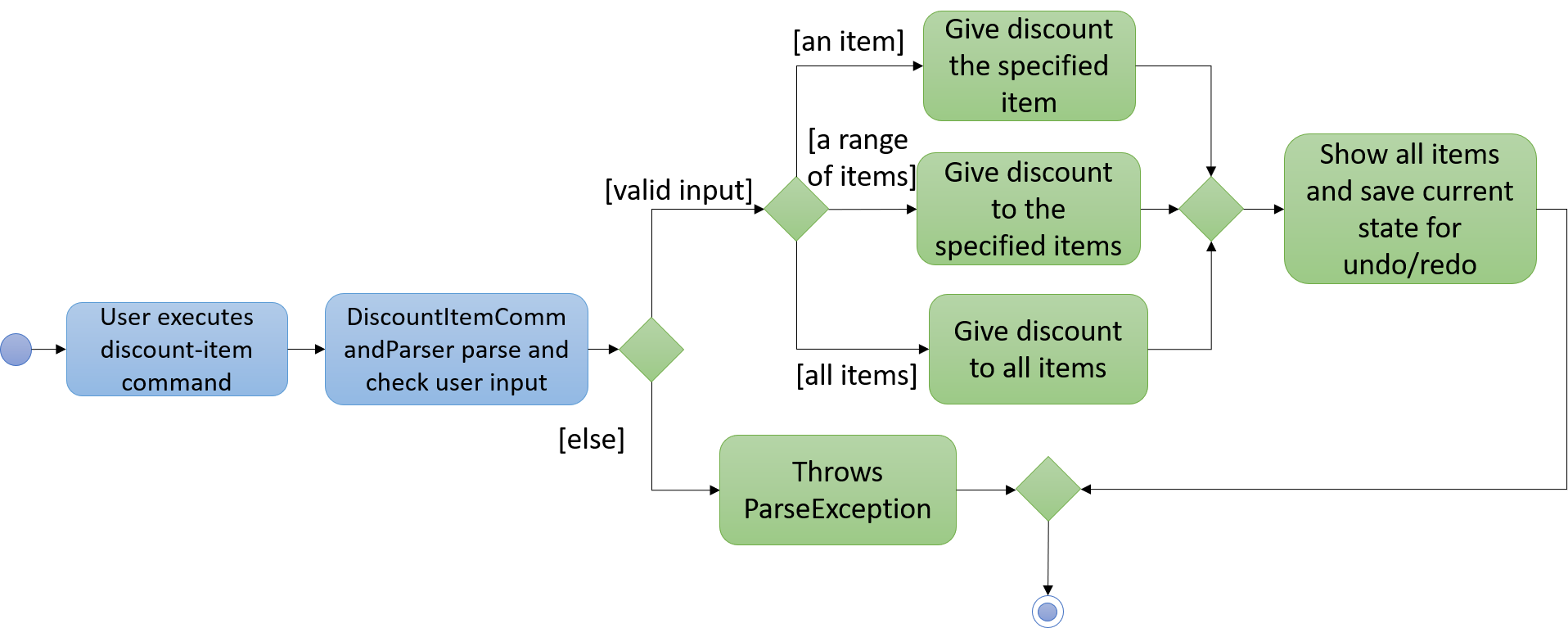
Figure 4.8.3.1: DiscountItem Activity diagram
Design Considerations
Aspect: How Price
is parsed
-
Alternative 1 (current choice):
Price
is parsed without the currency symbol.-
Pros: Easy to implement.
-
Cons: Only able to display
Price
with$
with 2 decimal place.
-
-
Alternative 2: Allow users to enter the currency symbol
-
Pros: Able to display the different currencies.
-
Cons: Harder to parse as currencies have different decimal places. Additional checks need to be implemented to check if the currency symbol and price entered are valid.
-
Extracts of Use Case Section
Use case: UC301 - Add item to menu
MSS
-
User requests to add item to menu.
-
App adds the item to menu.
-
App returns a success message confirming the new item has been added.
Use case ends.
Extensions
-
1a. Invalid argument given for the command.
-
1a1. App shows an error message that item name or/and item price are invalid.
-
1a2. User requests to add item to menu again.
Steps 1a1-1a2 are repeated until valid item name and valid item price are entered.
Use case resumes at step 2.
-
-
1b. The item name entered is already in the menu.
-
1b1. App shows an error message that the item name already exists.
-
1b2. User requests to add item to menu again.
Steps 1b1-1b2 are repeated until item name that does not exist in the menu is entered.
Use case resumes at step 2.
-
Use case: UC302 - Delete item by index from menu
MSS
-
User requests to list items.
-
App shows a list of items in menu.
-
User requests to delete a specific item in menu.
-
App deletes the item.
-
App returns a success message confirming the specified item has been deleted.
Use case ends.
Extensions
-
2a. The list is empty.
Use case ends.
-
3a. The given index is invalid.
-
3a1. App shows an error message that the given index is invalid.
-
3a2. User requests to delete a specific item in menu again.
Steps 3a1-3a2 are repeated until a valid index is entered.
Use case resumes at step 4.
-
Menu Management
-
Adding a new item with valid name, price and tag
-
Prerequisites: You must not have the item, specified in the test case below, in the menu.
-
Test case:
add-item n/Chicken Rice p/3 t/rice t/chicken
Expected: Item is added to the menu. Details of the item shown in status message. -
Incorrect commands to try:
add-item n/test p/string
,add-item n/@@^&@ p/3
-
-
Editing an item with valid name, price and tag
-
Prerequisites: You must have at least 1 item in the menu.
-
Test case:
edit-item 1 n/Duck Rice p/3 t/rice t/duck
Expected: Item specified by the index is updated with new details. New details of the item shown in status message. -
Incorrect commands to try:
edit-item 1 n/test p/string
,edit-item n/name p/3
-
-
Deleting an item by index
-
Prerequisites: You must have at least 1 item in the menu.
-
Test case:
delete-item-index 1
Expected: Item specified by the index is deleted from the menu. Number of items deleted shown in status message. -
Incorrect commands to try:
delete-item-index -2
,delete-item-index string
-
-
Deleting an item by name
-
Prerequisites: You must have the item, specified in the test case below, in the menu.
-
Test case:
delete-item-name chicken rice
Expected: Item specified by name is deleted from the menu. Details of the deleted item shown in status message. -
Incorrect commands to try:
delete-item-name @@^&@
-